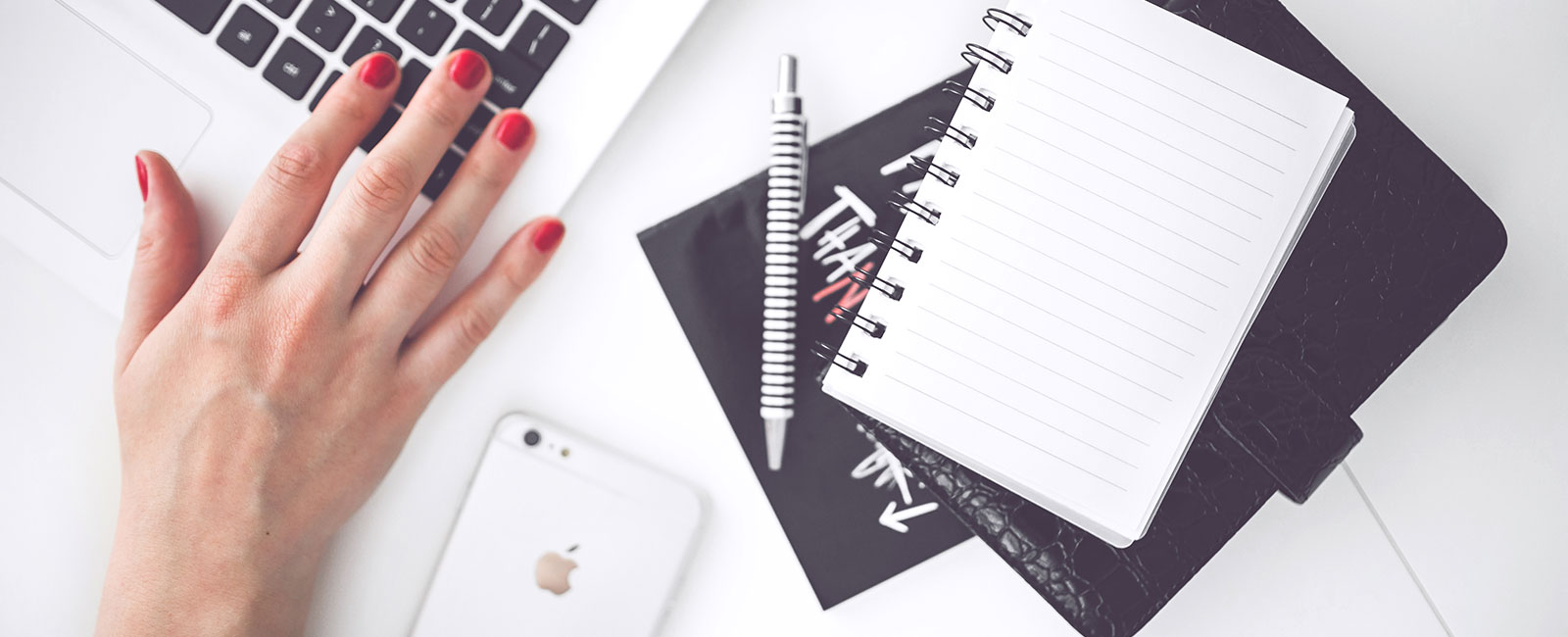
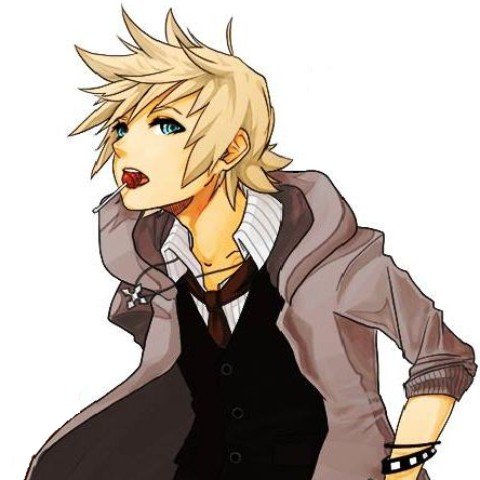
Mô tả bài toán
Tạo ứng dụng Console, sử dụng kiểu dữ liệuCấu trúc (Struct)
để lưu trữ thông tin Nhân viên trong công ty TNHH MTV SoftABC. Với yêu cầu:
Nhân viên phải được lưu trữ đầy đủ các thông tin sau:
- Họ tên
- Giới tính
- Ngày sinh, trong đó phải đầy đủ:
- Ngày sinh
- Tháng sinh
- Năm sinh
- Loại hợp đồng (Nhân viên Chính thức hay Nhân viên Tập sự)
- Có thể nhập liệu được Thông tin Nhân viên.
- Có thể In thông tin Nhân viên.
- Xem được tổng số Nhân viên đang có trong hệ thống là bao nhiêu? Tỉ lệ Nữ trong Cơ quan là bao nhiêu %?
- Tập sự đang có bao nhiêu Nhân viên? Chính thức có bao nhiêu Nhân viên?
- Ai là Nhân viên có số tuổi cao nhất? và nhỏ nhất?
Cách giải quyết
Ta sẽ sử dụng Cấu trúc (Struct) để lưu trữ thông tin Nhân viên như sau: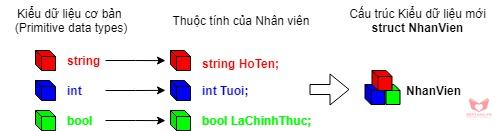
Source code
using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace StoreEmployeeUsingStruct { class Program { /// <summary> /// Cấu trúc Nhân viên bao gồm /// - Họ tên /// - Ngày sinh /// - Giới tính /// </summary> struct NhanVien { public string HoTen; public NgaySinhDataType NgaySinh; public int GioiTinh; public bool LaNhanVienChinhThuc; public int Tuoi; public void InThongTin() { Console.WriteLine("Ho ten: {0}", this.HoTen); Console.WriteLine("Ngay sinh: {0}", this.HoTen); Console.WriteLine("Tuoi: {0}", this.Tuoi); Console.WriteLine("Gioi tinh: {0}", (this.GioiTinh == 0) ? "Nam" : "Nu"); Console.WriteLine("Loai hop dong: {0}", (this.LaNhanVienChinhThuc == true) ? "Nhan vien chinh thuc" : "Nhan vien tap su"); } } /// <summary> /// Cấu trúc Ngày sinh bao gồm /// - Ngày /// - Tháng /// - Năm /// </summary> struct NgaySinhDataType { public int Day; public int Month; public int Year; } /// <summary> /// Dem tong so nu /// </summary> /// <param name="danhsachNhanVien"></param> /// <returns></returns> static int DemTongSoNu(NhanVien[] danhsachNhanVien) { int result = 0; for (int i = 0; i < danhsachNhanVien.Length; i++) { if(danhsachNhanVien[i].GioiTinh == 1) { result++; } } return result; } static NhanVien TimNhanVienTuoiCaoNhat(NhanVien[] danhsachNhanVien) { // Giả sử nhân viên đầu tiên tuổi cao nhất NhanVien temp = danhsachNhanVien[0]; // Duyệt danh sách các nhân viên đang có trong mảng // Nếu nhân viên nào cao tuổi hơn thì gán lại for (int i = 0; i < danhsachNhanVien.Length; i++) { if(danhsachNhanVien[i].Tuoi > temp.Tuoi) { temp = danhsachNhanVien[i]; } } return temp; } static void Main(string[] args) { int total = 3; Console.Write("\nNhap thong tin Nhan vien su dung Struct trong C#:\n"); Console.Write("--------------------------\n"); // Nhập thông tin danh sách [total] Nhân viên NhanVien[] emp = new NhanVien[total]; for (int i = 0; i < total; i++) { Console.WriteLine("--- NHAP THONG TIN NHAN VIEN THU {0} ---", (i+1)); // Họ tên Console.Write("Ho ten nhan vien: "); string hotenInput = Console.ReadLine(); emp[i].HoTen = hotenInput; // Nhập tuổi Console.Write("Nhap ngay sinh: "); int tuoiInput = Convert.ToInt32(Console.ReadLine()); emp[i].Tuoi = tuoiInput; // Giới tính Console.Write("Nhap gioi tinh (0: Nam; 1: Nu): "); int gioiTinhInput = Convert.ToInt32(Console.ReadLine()); emp[i].GioiTinh = gioiTinhInput; // Ngày tháng năm sinh Console.Write("Nhap ngay sinh: "); int ddInput = Convert.ToInt32(Console.ReadLine()); emp[i].NgaySinh.Day = ddInput; Console.Write("Nhap thang sinh: "); int mmInput = Convert.ToInt32(Console.ReadLine()); emp[i].NgaySinh.Month = mmInput; Console.Write("Nhap nam sinh: "); int yyInput = Convert.ToInt32(Console.ReadLine()); emp[i].NgaySinh.Year = yyInput; // Loại hợp đồng Console.Write("Nhap loai hop dong (Y: chinh thuc; N: tap su): "); string loaiHopDongInput = Console.ReadLine(); emp[i].LaNhanVienChinhThuc = (loaiHopDongInput == "Y") ? true : false; Console.WriteLine("--------------------------------------"); } // In thông tin danh sách [total] Nhân viên vừa nhập Console.WriteLine("=== DANH SACH NHAN VIEN ==="); for (int i = 0; i < total; i++) { Console.WriteLine("---Nhan vien thu {0}", (i+1)); emp[i].InThongTin(); Console.WriteLine("--------------------"); } Console.WriteLine("==========================="); // Dừng màn hình để kiểm tra Console.ReadKey(); } } }
Github
https://github.com/kellyfire611/learning.nentang.vn-csharp/blob/master/src/StoreEmployeeUsingStruct/Program.csChương trình học
Các bài học
Bài học trước Bài học tiếp theo
Chương trình học
Bao gồm Module, Chương, Bài học, Bài tập, Kiểm tra...Chương trình học
Bài học trước Bài học tiếp theo